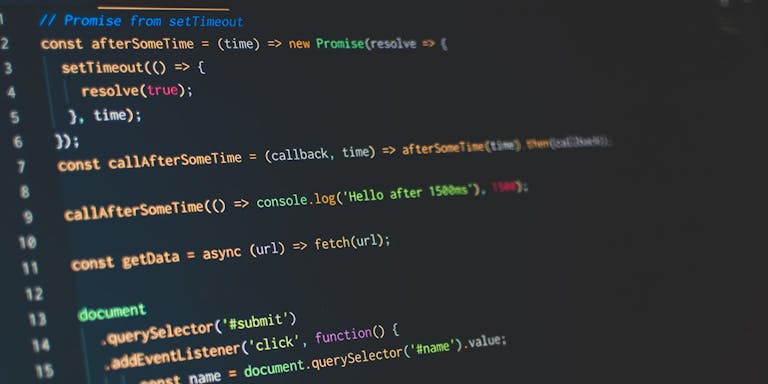
The difference between function scope and context in JavaScript
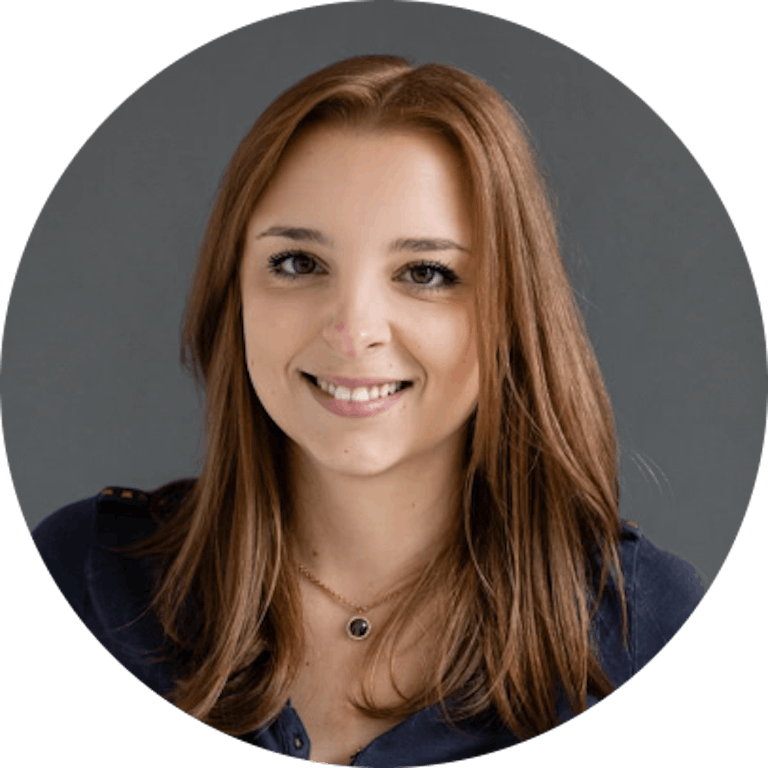
Jessica
April 8, 2019
JavaScript’s function scope and context can be very flexible and powerful, but also very confusing, especially for beginners. In this blog post I’m going to scratch the surface and explain the basic functionality of both concepts. The first thing to keep in mind is that scope and context are not the same.
Function scope
The scope determines the accessibility of variables and other resources in the code, like functions and objects. JavaScript function scopes can have two different types, the locale and the global scope. Local variables are declared within a function and can only be accessed within the function. Global variables are declared at top level outside any functions and all functions on a web page have access to them. Each function when invoked creates a new scope. While a global variable lives as long as your application lives, local variables are created when a function starts and deleted when no code has access to the variable anymore (usually when the function returns).
Global scope:
Local scope:
Function context
On the other hand there is the function context which can be accessed via this. It can have different values depending on how the function is invoked as well as using strict or non strict mode. Functions in non strict mode refer to the window object as context. Functions in strict mode refer to undefined.
When a function is called as a method of an object, the keyword this represents the value of the referenced object.
You are also able to change the context by using call(), apply() and bind() function methods. The methods call() and apply() execute the function with the given value as this. The only difference between those two is, that the passed arguments in the call() method are separated by a comma, while you pass the arguments for apply() as an array.
bind(), however, behaves differently and unlike call() and apply(), it doesn’t call the function itself. It creates a new function with the same body and scope as the original function but the context of the new function is bound to the first given argument of the bind() method.
This shows the basic functionality of how context and scope work and as a conclusion we can say that scope refers to the visibility of variables and context refers to this inside a function.
Jessica took an apprenticeship at Peerigon in 2019 to become a professional web developer. As part of her weekly routine, she publishes a blog post each week about what she has been doing and what she has learned.
JavaScript
Basics
Apprenticeship
Web Development
Tutorial
Read also
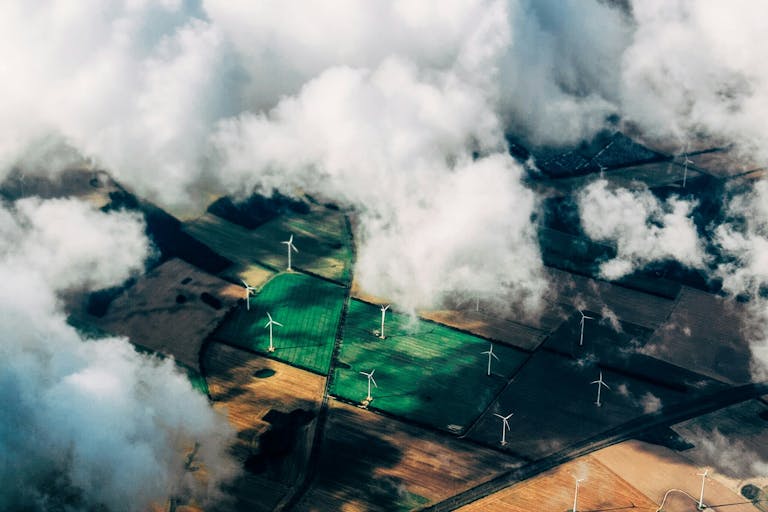
Michael, 04/16/2025
Green Hosting: A Sustainability Comparison
Sustainability
Green Software
Consulting
Hostingsec
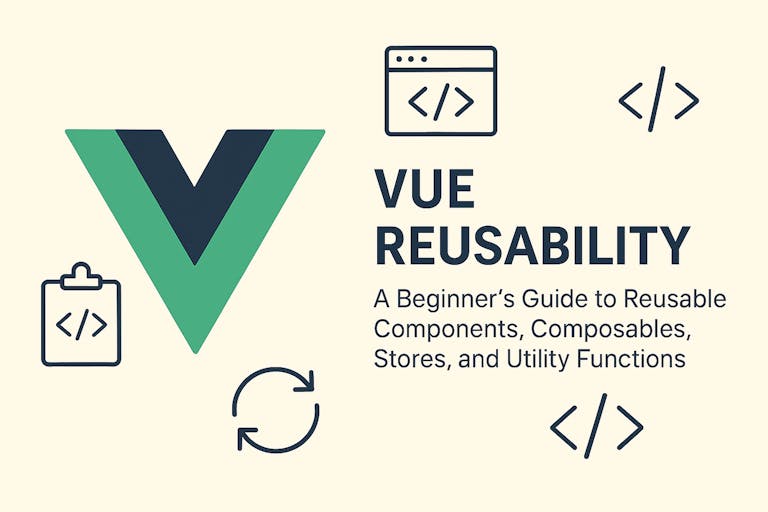
Lea, 04/04/2025
A Beginner's Guide to Reusability in Vue
Vue
JavaScript
Reusability
DRY Principle
Components

Francesca, Ricarda, 04/02/2025
Top 10 Mistakes to Avoid When Building a Digital Product
MVP development
UX/UI design
product vision
agile process
user engagement
product development